만들어볼 회원가입의 첫 번째 페이지는 다음과 같습니다.
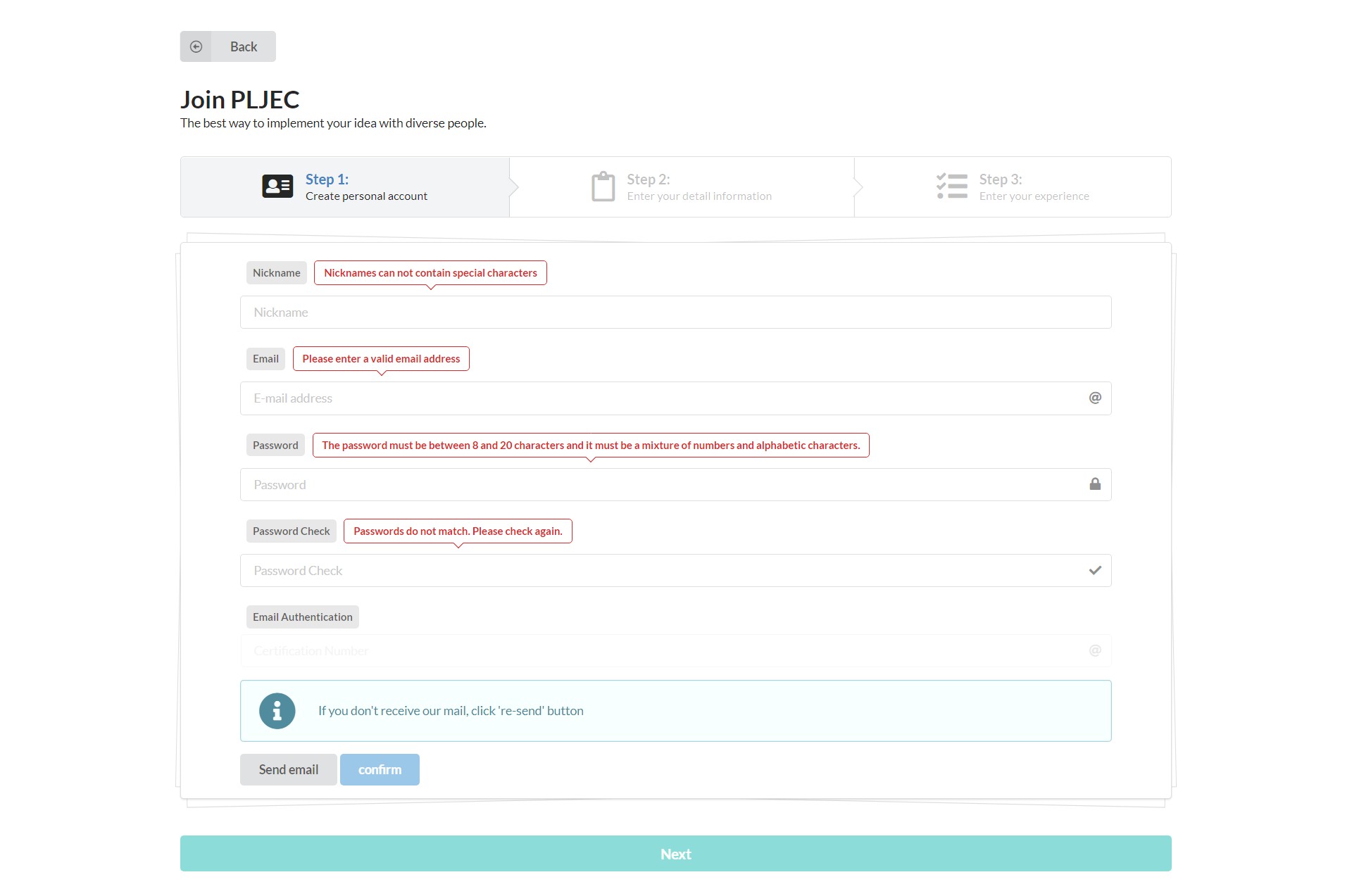
기본적으로 닉네임(아이디), 이메일, 비밀번호, 비밀번호 확인을 입력하는 양식이 있고 이메일 인증을 위한 요소들이 있습니다. 서버와의 연동은 나중에 생각하고 일단 동작하도록 하는 것이 목표입니다.
위 페이지는 다음과 같이 두 부분으로 나눌 수 있습니다.
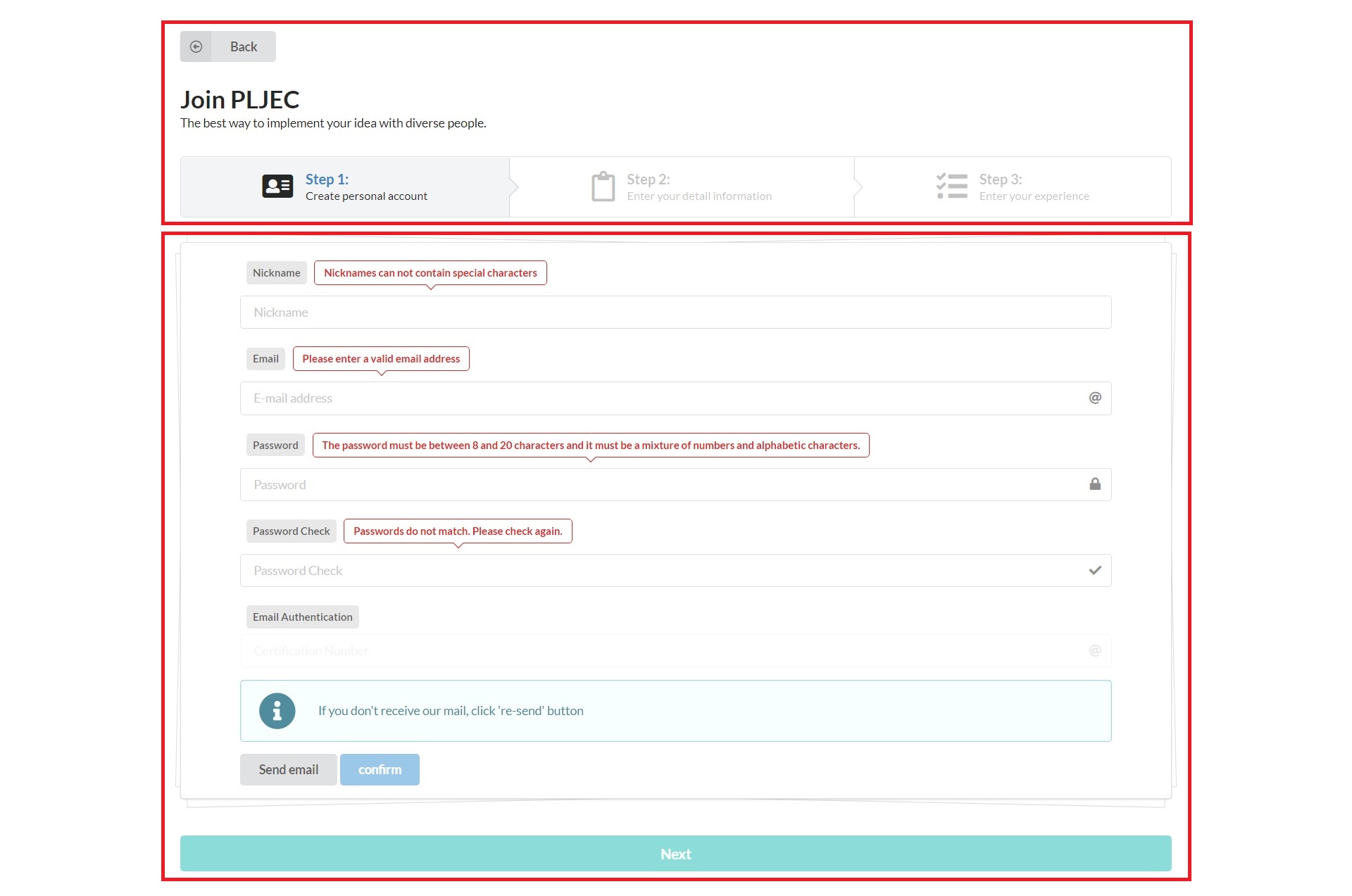
윗 부분은 3 페이지로 이루어진 회원가입 부분의 공통적으로 나타나게 되는 부분이고, 아래 부분은 각 페이지에서만 쓰이는 고유한 컴포넌트로 볼 수 있습니다.
먼저, 모든 회원가입 페이지에 공통적으로 사용되는 컴포넌트를 구현해보겠습니다.
components/Register/RegisterHeader.js
x
import React, {Component} from 'react';
import { Link } from 'react-router-dom';
import {
Button,
Container,
Step,
} from 'semantic-ui-react'
const style = {
h1: {
marginTop: '3em',
},
h2: {
margin: '4em 0em 2em',
},
h3: {
marginTop: '0.5em',
padding: '2em 0em',
},
last: {
marginBottom: '300px',
},
comp: {
margin:'0.5rem',
padding:'0.5rem'
},
base: {
marginBottom: '2rem',
}
}
class RegisterHeader extends Component {
render() {
const { number, onClickHome } = this.props;
const prior = (number > 1) ? '/signup/'+(number - 1) : '/';
return (
<div>
<Container style={style.h3}>
{ number !== '4' && <Link to={prior}><Button onClick={onClickHome} content='Back' icon='arrow alternate circle left outline' labelPosition='left' /></Link>}
<h1>Join PLJEC</h1>
The best way to implement your idea with diverse people.
</Container>
<Container style={style.base}>
<Step.Group fluid>
{ (number === '1' || number === undefined) ?
( <Step active icon='address card' title='Step 1:' description='Create personal account' /> )
: ( <Step disabled icon='address card' title='Step 1:' description='Create personal account' /> )
}
{ number === '2' ?
( <Step active icon='clipboard outline' title='Step 2:' description='Enter your detail information' /> )
: ( <Step disabled icon='clipboard outline' title='Step 2:' description='Enter your detail information' /> )
}
{ number === '3' ?
( <Step active icon='tasks' title='Step 3:' description='Enter your experience' /> )
: ( <Step disabled icon='tasks' title='Step 3:' description='Enter your experience' /> )
}
</Step.Group>
</Container>
</div>
);
};
}
export default RegisterHeader;
이 컴포넌트의 핵심 기능 두 가지는 페이지에 따라 Step이 선택되는 것과 이전 페이지로 돌아가는 기능입니다.
첫 번째 기능을 구현하기 위해서는 상위 컴포넌트인 페이지 컴포넌트로부터 페이지 번호를 전달받고, 삼항연산자를 사용하여 전달 받은 number
에 따라 active 상태의 Step 컴포넌트를 출력합니다.
x
<Step.Group fluid>
{ (number === '1' || number === undefined) ?
( <Step active icon='address card' title='Step 1:' description='Create personal account' /> )
: ( <Step disabled icon='address card' title='Step 1:' description='Create personal account' /> )}
{ number === '2' ?
( <Step active icon='clipboard outline' title='Step 2:' description='Enter your detail information' /> )
: ( <Step disabled icon='clipboard outline' title='Step 2:' description='Enter your detail information' /> )}
/* 생략 */
</Step.Group>
두 번째 기능도 역시 간단합니다. 전달 받은 number
를 통해 Link를 걸어주면 됩니다.
x
const prior = (number > 1) ? '/signup/'+(number - 1) : '/';
/* 생략 */
{ number !== '4' && <Link to={prior}><Button onClick={onClickHome} content='Back' icon='arrow alternate circle left outline' labelPosition='left' /></Link>}
/* 생략 */
이제 완성된 컴포넌트를 index.js에 추가해줍니다.
components/Register/index.js
/* 생략 */
export {default as RegisterHeader} from './RegisterHeader';
이제 페이지에 이 헤더를 추가해보겠습니다.
pages/Signup.js
xxxxxxxxxx
import React, {Component} from 'react';
import {PersonalInput} from 'components/Register';
import {DevelopInput} from 'components/Register';
import {ExperienceInput} from 'components/Register';
import {CompleteRegister} from 'components/Register';
import {RegisterHeader} from 'components/Register';
import {
Container,
} from 'semantic-ui-react'
class Signup extends Component {
render() {
const { match } = this.props;
const number = match.params.number;
return (
<div>
<RegisterHeader number={number} />
<Container style={{marginBottom: '2rem'}}>
{ (number === '1' || number === undefined) && <PersonalInput />}
{ (number === '2') && <DevelopInput />}
{ (number === '3') && <ExperienceInput/>}
{ (number === '4') && <CompleteRegister/>}
</Container>
</div>
)
}
};
export default Signup;
이제 실행시켜 회원가입 페이지를 들어가면 헤더가 나타나있는 것을 볼 수 있습니다.
http://localhost:3000/signup/ 뒤에 2, 3을 입력해보면 헤더가 페이지에 따라 올바르게 변경될 것 입니다.
본격적으로 개인정보 입력 양식 컴포넌트를 만들어보겠습니다.
component/Signup/PersonalInput.js
xxxxxxxxxx
import React, { Component } from 'react';
import { Link } from 'react-router-dom';
import {
Button,
Form,
Label,
Segment,
Message,
} from 'semantic-ui-react'
const style = {
base : {
margin:'0.5rem',
padding:'0.5rem'
},
paddinglr : {
paddingLeft : '6%',
paddingRight : '6%'
}
};
class PersonalInput extends Component {
render() {
return (
<div>
<Form>
<Segment piled style={style.paddinglr}>
<Label style={style.base}> Nickname </Label>
<Form.Input name='nickname' placeholder='Nickname'/>
<Label style={style.base}> Email </Label>
<Form.Input name='email' fluid icon='at' placeholder='E-mail address'/>
<Label style={style.base}> Password </Label>
<Form.Input name='password' fluid icon='lock' placeholder='Password' type='password'/>
<Label style={style.base}> Password Check </Label>
<Form.Input name='pcheck' fluid icon='check' placeholder='Password Check' type='password'/>
<Label style={style.base}> Email Authentication </Label>
<div>
<Form.Input name='certification_number' fluid icon='at' placeholder='Certification Number'/>
<Message style={{fontSize:'1rem'}} content="If you don't receive our mail, click 're-send' button" icon='info circle' info />
<Button> Send email </Button>
<Button primary>confirm</Button>
</div>
</Segment>
<Button disabled={true} color='teal' fluid size='large'>Next</Button>
</Form>
</div>
);
};
}
export default PersonalInput;
이제 회원가입의 첫 페이지가 완성된 것처럼 보입니다. 하지만 개인정보 입력 페이지에는 다음과 같은 기능들이 필요합니다.
- 올바르게 입력된 아이디인가?
- 이메일 주소 형식 확인
- 입력한 비밀번호 일치 확인
- 이메일 인증 기능
다음 글에서 상태 관리를 통해 위 기능들을 구현해보겠습니다.
'웹, 서버' 카테고리의 다른 글
[Ubuntu] 네트워크 연결 자동 재시도 (3) | 2020.04.22 |
---|---|
[Ubuntu] Ubuntu 설치 및 포맷 후 설정 총 정리 (+python 개발 환경) (0) | 2020.03.20 |
[Linux] 터미널에서 생성된 pyplot 그래프 보기 (1) | 2018.12.29 |
[Linux] no display name and no $DISPLAY environment variable 에러 해결 (0) | 2018.12.29 |
[Linux] 터미널 창 분할하기 (0) | 2018.12.29 |
댓글